Why you should use TypeScript for your frontend projects
Learn what makes TypeScript so good for your frontend projects and my top 3 use cases

Best reasons to use TypeScript for frontend projects
An extension of JavaScript adding type checking, TypeScript is becoming the new standard for frontend projects and for very good reasons.
Type Safety with static code analysis
TypeScript’s built-in programming type safety thanks to static code analysis helps detect errors early in the development workflow. You’ll either see them directly in place in your favorite IDE or they will be caught by your integration checks. This is “shifting-left” an entire class of bugs, preventing their impact on your business and reducing your overall maintenance costs.
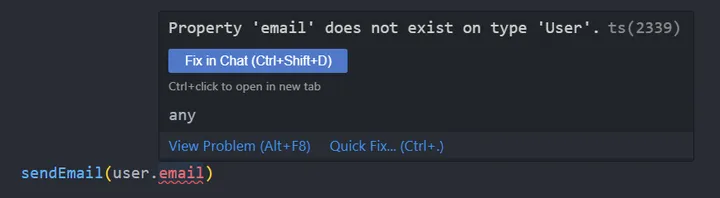
Enhanced Developer Experience
Even though you could achieve similar results with JavaScript and a good IDE, TypeScript’s integration with IDEs and tooling is superior to none and will speed up your development workflow with the likes of autocompletion and auto imports.
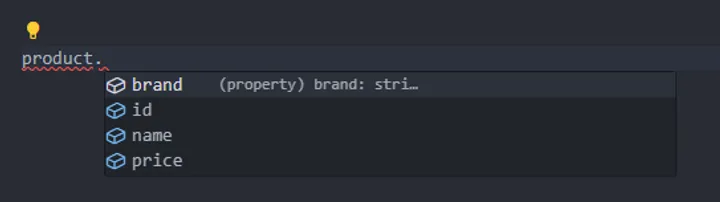
Tools and Ecosystem integration
TypeScript is now a first-class citizen in the JavaScript ecosystem, especially considering that Node.js recently added support to run TypeScript code natively.
Since Node.js version 22.6.0 where you can run TypeScript code directly with Node.js using the --experimental-strip-types
flag:
node --experimental-strip-types index.ts
And from version 23 onwards, this flag is enabled by default and you can run the following command to execute a TypeScript file:
node index.ts
Note that this only works for code that do not require transformations as described in the Node.js documentation.
What is also great is that you can gradually adopt TypeScript in your project by keeping your existing JavaScript codebase and adding TypeScript to new parts.
TypeScript is also one of the most popular languages to work with LLMs and AI agents. This is not surprising given its advantages on developer experience with built-in documentation for methods parameters and return types.
My top 3 use cases for TypeScript
- Safe refactorings
When I am refactoring code used in multiple places such as UI components, TypeScript helps me find all the places that need to be updated.
Let’s suppose I have a UI component that exposes a title
prop which type was
title?: string
(meaning it was optional). I have later realized that this component should not be used without a title and I want to enforce this. This is a breaking change and in a very large codebase, it could be a time consuming task to find all the places that need to be updated.
When running TypeScript locally or in my CI pipeline, it will list all the places that are now breaking and need to be updated:
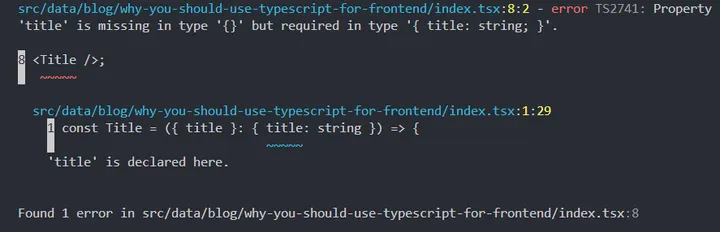
- End-to-end type safety with your APIs
After static analysis type safety, this is probably my favorite use case for TypeScript. When you are integrating a frontend application with an API, you can generate TypeScript types from the API specification to use in your frontend application.
There are many packages available to help you generate types from an API specification and I can recommend OpenAPI TypeScript.
I created a small demo repository to show you how to use the generated types in your frontend application.
Not only does it speed up your development workflow with in code documentation and autocompletion but it will also prevent you from misuing the API which usually leads to bugs in your application.
The code autocompletion from this kind of setup is also fantastic and will save you a lot of time.
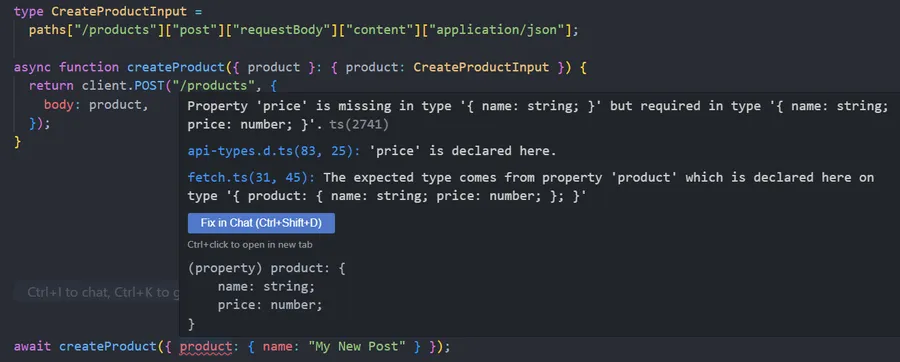
- Inline documentation for packages
The last use case I want to cover is the ability it gives you to have documentation directly from your IDE when using third-party packages. I know JSDoc can help you achieve the same, in fact it can also complement your TypeScript code but I find it more cumbersome to use for simple cases.
Below is an example of how TypeScript helped me configure the client for openapi-fetch
.
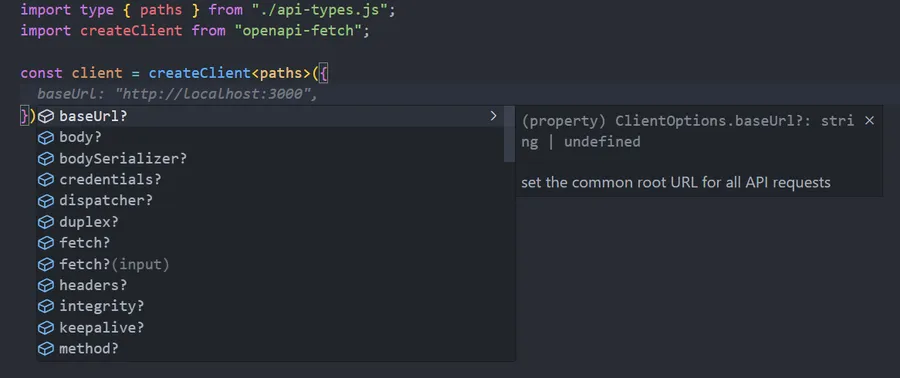
It will also make your life easier when building your own packages as TypeScript will help you generate types to use for your end users.
You can find more information on how to get started with TypeScript and how to migrate a large codebase to TypeScript in my documentation site.
If you were not already convinced on TypeScript, I hope you are now!